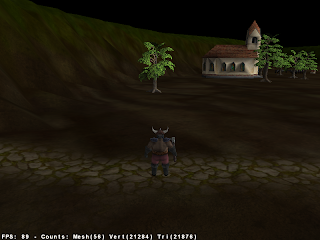
I've been playing with JMonkey for the last 2 years at least and for the current project I'm using a data-driven aproach. For those who don't know what this strange term is, data-driven means: reusable plug-and-play components you can attach to your game with declarative or script languages.
In my engine I'm using XML to define types, objects and gamestates, but this requires me explanation. GameStates enable you to encapsulate different scene concepts (or scene graphs) like: gameplay scene, inGame menus, main menus, each in its own state object. This aproach makes it easy to switch between them by enabling and disabling these GameState objects.
My idea is to define a Game as a collection of GameStates, each one composed by a collection of GameObject instances. Each of these GameObject instances is by itself a collection of pluggable Components. This GameObject design is based on an article from the book Game Programming Gems 6 - "Game Object Component System" - by Chris Stoy. One can find useful information in this post as well, by Robert Rose.
A GameObject can be anything like a player, with a 3D model, a static scene element or even a positional trigger of some type, it doesn't matter, all of them are just this: GameObjects with a collection of Components defining their looks and behaviors.
The goal is to develop fine grained reusable Components that can be combined in ways to express the desired game design and behavior. I've already developed a lot of these reusable components, some independent and some dependent on other to work:
- VisualComponent - defines a 3D model to be imported, scaled, rotated and tranlated;
- PhysicsComponent - plugs a static physics behavior for a GameObject with a VisualComponent;
- DynamicPhysicsComponent - same as above, but with mass and dynamic physics;
- PositionalAudioComponent - allows the attachment of a positional audio clip in declarative form;
- TemporalTrigger - enables and disables child Components based on a timer;
- TerrainComponent - permits the declaration of heightmap based terrain;
- TerrainPhysics - for attaching physics to the terrain;
- TerrainTexture - for composing and glueing splatts, color and detail textures.
Last but not least: You can think the game architecture as a big mediator (design pattern) between gamestates switchs (gamestates defined as collection of game objects in XML). GameObjects and Components implement the Composite design pattern since both can have collection of Components. The XML definitions use the concepts of inheritance. One can define a generic type, with a collection of components and refine their attributes in inherited declarations.
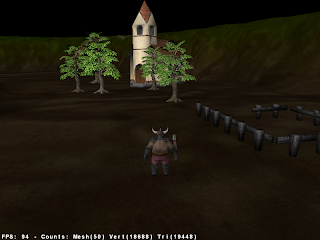
Multiple Components of the same type are allowed inside the same collection, the order of declaration is guaranteed in initialization and I used a LOT of reflection in the parsing and gamestates building fases. Too much blah blah blah? So lets see some action:
Definition of a "game":
<game name="Sertao 3D" init="caatinga">
<!-- PATHS FOR RESOURCE LOCATOR -->
<path dir="/sample/resource" type="texture">
<path dir="/sample/resource" type="model">
<!-- TYPE DEFINITIONS -->
<include file="/sample/types-basic.xml">
<include file="/sample/types-terrain.xml">
<include file="/sample/types-player.xml">
<include file="/sample/types-misc.xml">
<!-- GAMESTATES -->
<include file="/sample/caatinga.xml">
</game>
The abouve XML defines a game by including type-definitions and one gamestate. Lets see some samples of them:
Extract from types-player.xml:
<type name="player">
<component class="gcore.component.VisualComponent">
<model value="./sample/resource/dwarf.jme">
<scale x="0.03" y="0.03" z="0.03">
<rotation y="-90">
</component>
<component class="gcore.component.DynamicPhysicsComponent">
<mass value="2">
<position y="2">
</component>
<component class="gcore.component.PlayerCameraComponent">
<offset y="4" z="8">
</component>
<component class="component.ControllerComponent">
<speed value="3.5">
</component>
</type>
This shows a player as being a 3D model, with physics behavior, followed by the camera and controlled by an especialized controller. To expose more lets see a n inheritance example with two extracts:
From types-basic.xml:
<type name="visualPhysics">
<component class="gcore.component.VisualComponent">
<component class="gcore.component.PhysicsComponent">
<triangleaccurate value="true">
</component>
</type>
And from types-misc.xml:
<type name="oldfence" extends="visualPhysics">
<component class="gcore.component.VisualComponent">
<model value="./sample/resource/oldfence.jme">
</component>
<component class="gcore.component.PositionalAudioComponent">
<file value="/sample/resource/audio/cricket2.wav">
<playrange value="25">
<stoprange value="25">
<maxvolume value="0.15">
</component>
</type>
As you can see, the oldfence type inherits the components and attributes from the visualPhysics one and spectializes some things, including a positional audio clip and using a specific 3d model.
How can we use this in a GameState? An extract from the gamestate definition file caating.xml:
<object name="fence1" extends="oldfence">
<component class="gcore.component.VisualComponent">
<position x="40" z="10">
</component>
</object>
As you can see, we just need to overhide the specific position information for the visual model and that's it. You can download the entire source (zipped eclipse project) for the engine and the above example at this link.
Any comments are welcome.
5 comments:
O Blog foi muito boa idéia. E esse livro sobre JMonkey? não sabia dessa daí. Já tem algum outro?
Não existe nenhum ainda.
[English]
There's no JMonkey book yet. I've been talking to the engine developers and they fully support the idea.
The code link is broken. Fix it please, I'm looking forward to it. It seems a great idea.
Would be great if you shared you code, link is broken -- thanks, I read your paper, it was very insightful.
Hello! Zelda Niblett . payday loans
Post a Comment